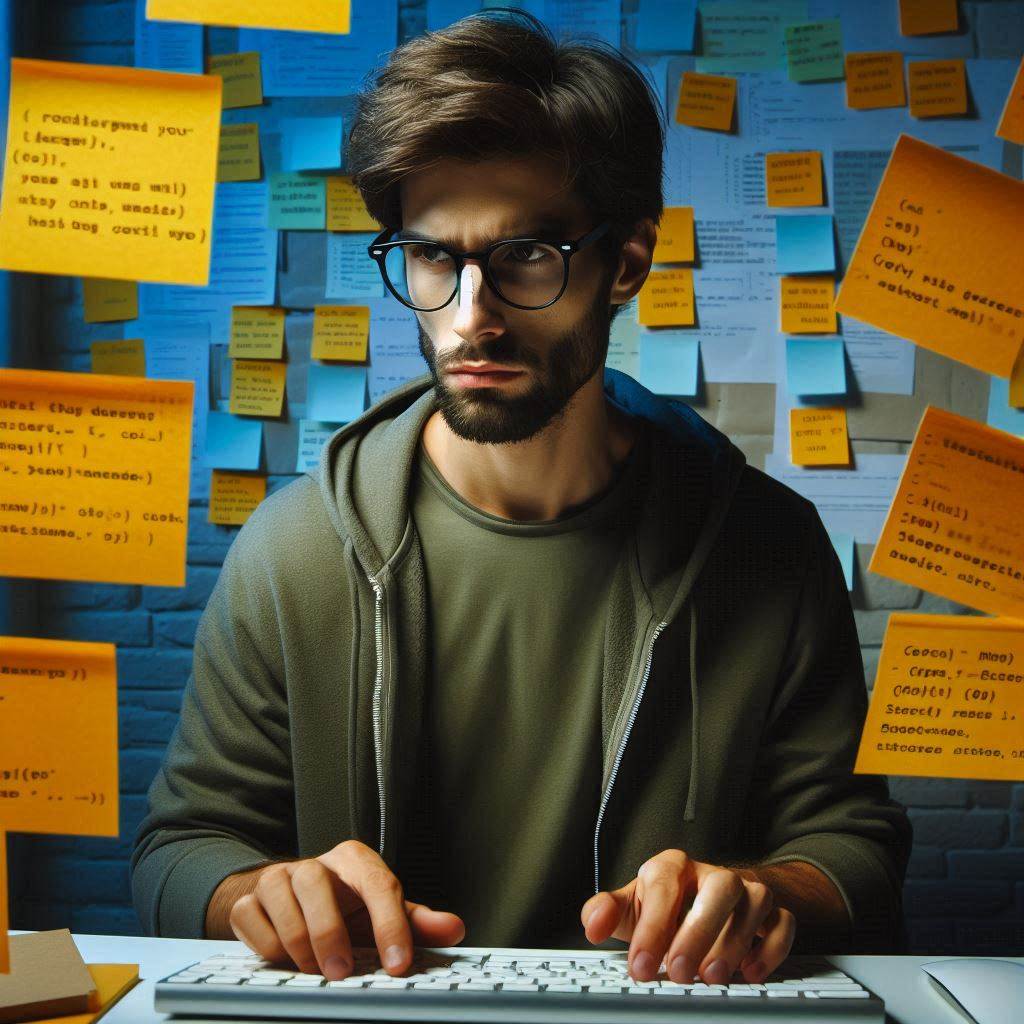
Laravel Examples
Dependency Injection
PHP
// app/Services/EmailService.php
class EmailService
{
public function send(string $to, string $subject, string $message)
{
// Send email using a third-party library
// ...
}
}
// app/Http/Controllers/UserController.php
class UserController extends Controller
{
public function __construct(EmailService $emailService)
{
$this->emailService = $emailService;
}
public function register(Request $request)
{
// ...
$this->emailService->send($request->input('email'), 'Welcome Email', 'Thank you for registering!');
// ...
}
}
Explanation: The EmailService
is injected into the UserController
as a dependency, ensuring loose coupling and testability.
Facades
PHP
// app/Http/Controllers/PostController.php
class PostController extends Controller
{
public function index()
{
$posts = DB::table('posts')->get();
return view('posts.index', compact('posts')); <a target="_blank" rel="noreferrer noopener" href="https://github.com/JaberSalama/Query_builder"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/JaberSalama/Query_builder"> 1. github.com </a><a target="_blank" rel="noreferrer noopener" href="https://github.com/JaberSalama/Query_builder"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/JaberSalama/Query_builder"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/JaberSalama/Query_builder"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/JaberSalama/Query_builder">github.com</a><a target="_blank" rel="noreferrer noopener" href="https://github.com/JaberSalama/Query_builder"></a>
}
}
Explanation: The DB
facade provides a convenient way to interact with the database.
Middleware
PHP
// app/Http/Middleware/AuthMiddleware.php
class AuthMiddleware
{
public function handle($request, Closure $next)
{
if (!Auth::check()) {
return redirect('/login');
}
return $next($request); <a target="_blank" rel="noreferrer noopener" href="https://github.com/nguyenthutuyet30/Arome"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/nguyenthutuyet30/Arome"> 1. github.com </a><a target="_blank" rel="noreferrer noopener" href="https://github.com/nguyenthutuyet30/Arome"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/nguyenthutuyet30/Arome"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/nguyenthutuyet30/Arome"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/nguyenthutuyet30/Arome">github.com</a><a target="_blank" rel="noreferrer noopener" href="https://github.com/nguyenthutuyet30/Arome"></a>
}
}
Explanation: The AuthMiddleware
checks if the user is authenticated and redirects to the login page if not.
Routing
PHP
// routes/web.php
Route::get('/', 'HomeController@index');
Route::get('/posts', 'PostController@index');
Route::post('/posts', 'PostController@store');
Explanation: Routes map URLs to specific controller methods.
Controllers
PHP
// app/Http/Controllers/PostController.php
class PostController extends Controller
{
public function index()
{
$posts = Post::all();
return view('posts.index', compact('posts'));
}
public function store(Request $request) <a target="_blank" rel="noreferrer noopener" href="https://stackoverflow.com/questions/67210803/laravel-8-uploading-files-using-ajax"></a><a target="_blank" rel="noreferrer noopener" href="https://stackoverflow.com/questions/67210803/laravel-8-uploading-files-using-ajax"> 1. stackoverflow.com </a><a target="_blank" rel="noreferrer noopener" href="https://stackoverflow.com/questions/67210803/laravel-8-uploading-files-using-ajax"></a><a target="_blank" rel="noreferrer noopener" href="https://stackoverflow.com/questions/67210803/laravel-8-uploading-files-using-ajax"></a><a target="_blank" rel="noreferrer noopener" href="https://stackoverflow.com/questions/67210803/laravel-8-uploading-files-using-ajax"></a><a target="_blank" rel="noreferrer noopener" href="https://stackoverflow.com/questions/67210803/laravel-8-uploading-files-using-ajax">stackoverflow.com</a><a target="_blank" rel="noreferrer noopener" href="https://stackoverflow.com/questions/67210803/laravel-8-uploading-files-using-ajax"></a>
{
$post = Post::create($request->all());
return redirect()->route('posts.show', $post);
}
}
Explanation: Controllers handle incoming requests and return responses.
Eloquent ORM
PHP
// app/Models/Post.php
class Post extends Model
{
protected $fillable = ['title', 'content'];
public function comments()
{
return $this->hasMany(Comment::class);
}
}
Explanation: Eloquent provides a convenient way to interact with database tables.
Blade Templating
HTML
@extends('layouts.app')
@section('content')
<h1>Posts</h1>
<ul>
@foreach ($posts as $post)
<li>{{ $post->title }}</li>
@endforeach
</ul>
@endsection
Explanation: Blade templates allow you to create dynamic views using PHP-like syntax.
Artisan CLI
Bash
php artisan make:controller PostController
php artisan migrate
php artisan serve
Explanation: Artisan is a command-line interface for Laravel.
Node.js Examples
Asynchronous Programming
JavaScript
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Explanation: The readFile
method is asynchronous, and the callback function is executed when the operation completes.
Modules
JavaScript
const http = require('http');
const server = http.createServer((req, res) => {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!');
});
server.listen(3000, <a target="_blank" rel="noreferrer noopener" href="https://www.yisu.com/zixun/596366.html"></a><a target="_blank" rel="noreferrer noopener" href="https://www.yisu.com/zixun/596366.html"> 1. www.yisu.com </a><a target="_blank" rel="noreferrer noopener" href="https://www.yisu.com/zixun/596366.html"></a><a target="_blank" rel="noreferrer noopener" href="https://www.yisu.com/zixun/596366.html"></a><a target="_blank" rel="noreferrer noopener" href="https://www.yisu.com/zixun/596366.html"></a><a target="_blank" rel="noreferrer noopener" href="https://www.yisu.com/zixun/596366.html">www.yisu.com</a><a target="_blank" rel="noreferrer noopener" href="https://www.yisu.com/zixun/596366.html"></a> () => {
console.log('Server listening on port 3000');
});
Explanation: The http
module is used to create an HTTP server.
npm
Bash
npm install express
Explanation: The npm
command is used to install the express
package.
Event-Driven Programming
JavaScript
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on('event', () => {
console.log('An event occurred!');
});
myEmitter.emit('event');
Explanation: Event emitters and listeners are used to handle events in Node.js.
HTTP Requests and Responses
JavaScript
const http = require('http');
const server = http.createServer((req, res) => {
if (req.url === '/hello') {
res.writeHead(200, {'Content-Type': 'text/plain'});
res.end('Hello, World!');
} else {
res.writeHead(404);
res.end('Not <a target="_blank" rel="noreferrer noopener" href="https://github.com/AmanSilawat/Course--Digging-Into-Node.js"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/AmanSilawat/Course--Digging-Into-Node.js"> 1. github.com </a><a target="_blank" rel="noreferrer noopener" href="https://github.com/AmanSilawat/Course--Digging-Into-Node.js"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/AmanSilawat/Course--Digging-Into-Node.js"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/AmanSilawat/Course--Digging-Into-Node.js"></a><a target="_blank" rel="noreferrer noopener" href="https://github.com/AmanSilawat/Course--Digging-Into-Node.js">github.com</a><a target="_blank" rel="noreferrer noopener" href="https://github.com/AmanSilawat/Course--Digging-Into-Node.js"></a> Found');
}
});
Explanation: The http
module is used to handle HTTP requests and responses. Sources and related content