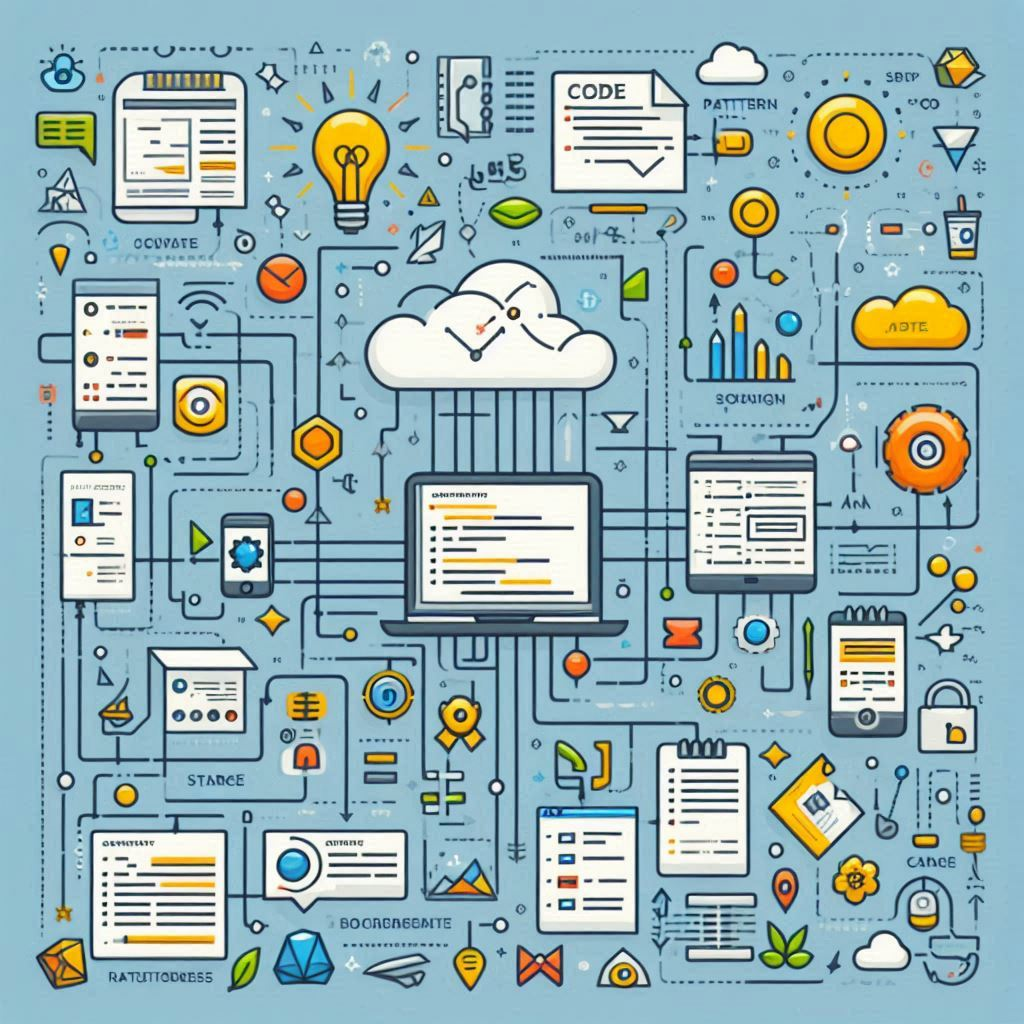
Design patterns are reusable solutions to common software design problems. They provide a proven framework for creating flexible, scalable, and maintainable code. There are three main categories of design patterns:
1. Creational Patterns
These patterns focus on object creation mechanisms. They provide ways to create objects flexibly, without specifying their concrete classes.
- Factory Method: Defines an interface for creating an object, but lets subclasses decide which class to instantiate.
- Abstract Factory: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Prototype: Creates new objects by copying an existing object.
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
2. Structural Patterns
These patterns deal with how classes and objects are composed to form larger structures. They focus on relationships between objects.
- Adapter: Converts the interface of a class into another interface clients expect.
- Bridge: Decouples an abstraction from its implementation, so the two can vary independently.
- Composite: Composes objects into tree structures to represent part-whole hierarchies.
- Decorator: Adds responsibilities to objects dynamically.
- Facade: Provides a unified interface to a set of interfaces in a subsystem.
- Flyweight: Shares common objects to reduce memory usage.
- Proxy: Provides a surrogate or placeholder for another object to control access to it.
3. Behavioral Patterns
These patterns concern how objects interact and communicate with each other. They address algorithmic and responsibilities between objects.
- Chain of Responsibility: Avoids coupling the sender of a request to its receiver by giving more than one object a chance to handle the request.
- Command: Encapsulates a request as an object, thereby letting you parameterize clients with different requests, queue or log requests, and support undoable operations.
- Interpreter: Defines a grammar for a language and provides an interpreter to evaluate sentences in the language.
- Iterator: Provides a way to access the elements of an aggregate object sequentially without exposing its internal representation.
- Mediator: Defines an object that encapsulates how a set of objects interact. The mediator promotes loose coupling by keeping objects from referring to each other directly.
- Memento: Captures and restores an object’s internal state so that it can be returned to this state later.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- State: Allows an object to alter its behavior when its internal state changes. The object will appear to change its class.
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable. Strategy lets the algorithm vary independently from clients that use it.
- Template Method: Defines the skeleton of an algorithm in an operation, deferring some steps to subclasses. Template Method lets subclasses redefine certain steps of an algorithm without changing the algorithm’s structure.
- Visitor: Represents an operation to be performed on the elements of an object structure. Visitor lets you define new operations without changing the classes of the elements that are visited.
4. Some additional patterns you might encounter include
Anti-Patterns: These are common design practices that are often ineffective or harmful. Recognizing and avoiding anti-patterns is essential for good software design.
Architectural Patterns: These patterns focus on the overall structure of a system, such as Model-View-Controller (MVC), Layered, and Microservices.
Concurrency Patterns: Designed for handling concurrent operations and avoiding issues like deadlocks and race conditions.