Single Responsibility Principle
The single responsibility principle (SRP) is a fundamental concept in software design. It states that a class, module, or function should have one, and only one, reason to change.
In simpler terms, each part of your code should have a single, well-defined purpose. This makes your code easier to understand, maintain, and modify.
Here’s a breakdown of the SRP:
- Focus on a single responsibility: A class or function should handle one specific task or set of related tasks.
- Reason to change: If there’s more than one reason a piece of code might need to be changed, it’s likely violating the SRP.
Here’s an example:
Imagine a class called Invoice
that handles creating invoices, calculating totals, and sending them via email. This class violates SRP because it has three distinct responsibilities:
- Invoice creation
- Calculation
- Email communication
Following SRP, these functionalities would be separated into different classes:
Invoice
class: Handles creating invoices and calculating totals.EmailService
class: Handles sending emails.
By following SRP, your code becomes more modular, reusable, and easier to test and debug.
Open-Closed Principle
The open-closed principle (OCP) is another important principle in object-oriented design (OOP). It states that software entities (classes, modules, functions, etc.) should be:
- Open for extension: You should be able to add new functionalities to the existing code without modifying the original code itself.
- Closed for modification: The core functionality of the existing code should be protected and not changed when adding new features.
This might seem contradictory at first, but it basically means your code should be designed in a way that allows for future growth without breaking what already works.
Here’s a breakdown of the OCP:
- Benefits of extension:
- Easier to add new features without rewriting existing code.
- Promotes code reusability.
- Closed for modification:
- Reduces the risk of introducing bugs when adding new features.
- Protects the core functionality from unintended changes.
OCP is often achieved through techniques like:
- Abstraction: Using interfaces or abstract classes to define the functionalities that can be extended.
- Inheritance: Creating subclasses that inherit the core functionality and add new behaviors.
- Dependency injection: Injecting dependencies into your code to allow for flexible behavior.
Here’s an example:
Imagine you have a program that calculates shapes’ areas. Initially, it might only support squares and circles. Following OCP, you could define an interface for calculating area and have separate classes for squares and circles implementing that interface. This allows you to easily add new shapes (e.g., triangles) in the future without modifying the existing code for squares and circles.
By following OCP, you create more maintainable, adaptable, and future-proof code.
Liskov Substitution Principle
The Liskov Substitution Principle (LSP) is a cornerstone of object-oriented design that ensures your code is robust and flexible. Here’s how it works:
Core principle: Objects of a superclass should be interchangeable with objects of its subclasses without affecting the program’s correctness.
Imagine a superclass called Animal with a method eat(). LSP dictates that any subclass of Animal, like Dog or Bird, should have their own eat() method that behaves in a way consistent with the general concept of eating for animals.
Benefits:
Reliable Code: By ensuring subclasses adhere to the superclass’s expectations, you prevent unexpected behavior when using subclasses.
Maintainable Code: LSP promotes loose coupling, where code relies on interfaces or abstract superclasses, making it easier to modify or extend functionality without breaking existing code.
Avoiding Violations:
Stricter Preconditions: A subclass shouldn’t have stricter requirements for using its methods compared to the superclass. For instance, if Animal.eat() accepts any food, a Dog.eat() shouldn’t expect only a specific type of dog food.
Weaker Postconditions: A subclass shouldn’t guarantee less than what the superclass guarantees. If Animal.eat() reduces hunger level, a Bird.eat() shouldn’t leave the bird hungrier.
Example (Violation):
Imagine a Rectangle class inherits from a Shape class with a calculateArea() method. If Shape.calculateArea() throws an error for non-rectangular shapes, but Rectangle.calculateArea() always returns a value, substituting a Rectangle for a general Shape might lead to errors where the program expects an exception.
By following LSP, you create a hierarchy of classes where subclasses are truly extensions of their superclasses, promoting robust and maintainable object-oriented code.
Interface Segregation Principle
The Interface Segregation Principle (ISP) is one of the SOLID principles of object-oriented design. It focuses on how interfaces are designed and used. Here’s the core idea:
- Clients shouldn’t depend on methods they don’t use: A class or piece of code shouldn’t be forced to implement functionalities (methods) that it doesn’t need.
This principle promotes creating smaller, more specific interfaces instead of large, generic ones. This leads to several advantages:
- Reduced Coupling: Classes only depend on the functionalities they actually use. This makes the code more loosely coupled and easier to manage.
- Improved Maintainability: Smaller interfaces are easier to understand, modify, and test.
- Flexibility: New functionalities can be added by creating new, specific interfaces without affecting existing code.
Here’s an analogy: Imagine a gym membership. An ideal scenario would be to have different memberships for different needs. Someone who only wants to use the weights wouldn’t have to pay for access to the pool they don’t use. ISP is similar – classes “pay” only for the functionalities they actually “use.”
Example:
Let’s say you have an interface named PaymentProcessor
with methods for credit card payments, bank transfers, and PayPal payments. A class for processing cash payments wouldn’t need to implement the credit card or PayPal methods. This violates ISP.
Following ISP, you could create separate interfaces:
CreditCardProcessor
for credit card paymentsBankTransferProcessor
for bank transfersCashPaymentProcessor
for cash payments (with no unnecessary methods)
This way, classes only implement the functionalities they need, making the code cleaner and more maintainable.
Dependency Inversion Principle
The Dependency Inversion Principle (DIP) is another one of the core SOLID principles in object-oriented design. It focuses on how dependencies are established between different parts of your code. Here’s the key concept:
- High-level modules shouldn’t depend on low-level modules. Both should depend on abstractions.
Imagine a high-level module like an “engine” and a low-level module like a “specific fuel pump.” DIP suggests that the engine shouldn’t depend on the specific fuel pump implementation. Instead, they should both rely on an abstraction like a “FuelProvider” interface. This interface defines the functionality the engine needs (providing fuel), without specifying how it’s achieved.
Here’s a breakdown of the benefits of DIP:
- Loose Coupling: By depending on abstractions, modules become loosely coupled. This makes them more independent and easier to test, modify, and reuse.
- Flexibility: You can easily swap out concrete implementations (like different fuel pumps) without affecting the high-level module (engine) as long as they adhere to the abstraction (FuelProvider).
- Maintainability: Code becomes more maintainable as changes in low-level modules don’t ripple through the entire system.
Here’s an analogy: Think of building a house. The house (high-level module) depends on the functionality of doors and windows (abstractions) but not on specific brands or models (low-level modules). You can replace doors and windows with different ones as long as they fulfill the functionalities defined by the abstractions.
Dependency Inversion vs Dependency Injection:
DIP is a principle, while Dependency Injection (DI) is a technique to achieve DIP. DI involves injecting dependencies (like the FuelProvider) into the high-level module (engine) instead of creating them directly within the module. This allows for more control over dependencies and further promotes loose coupling.
By following DIP, you create more robust, adaptable, and easier-to-maintain code.
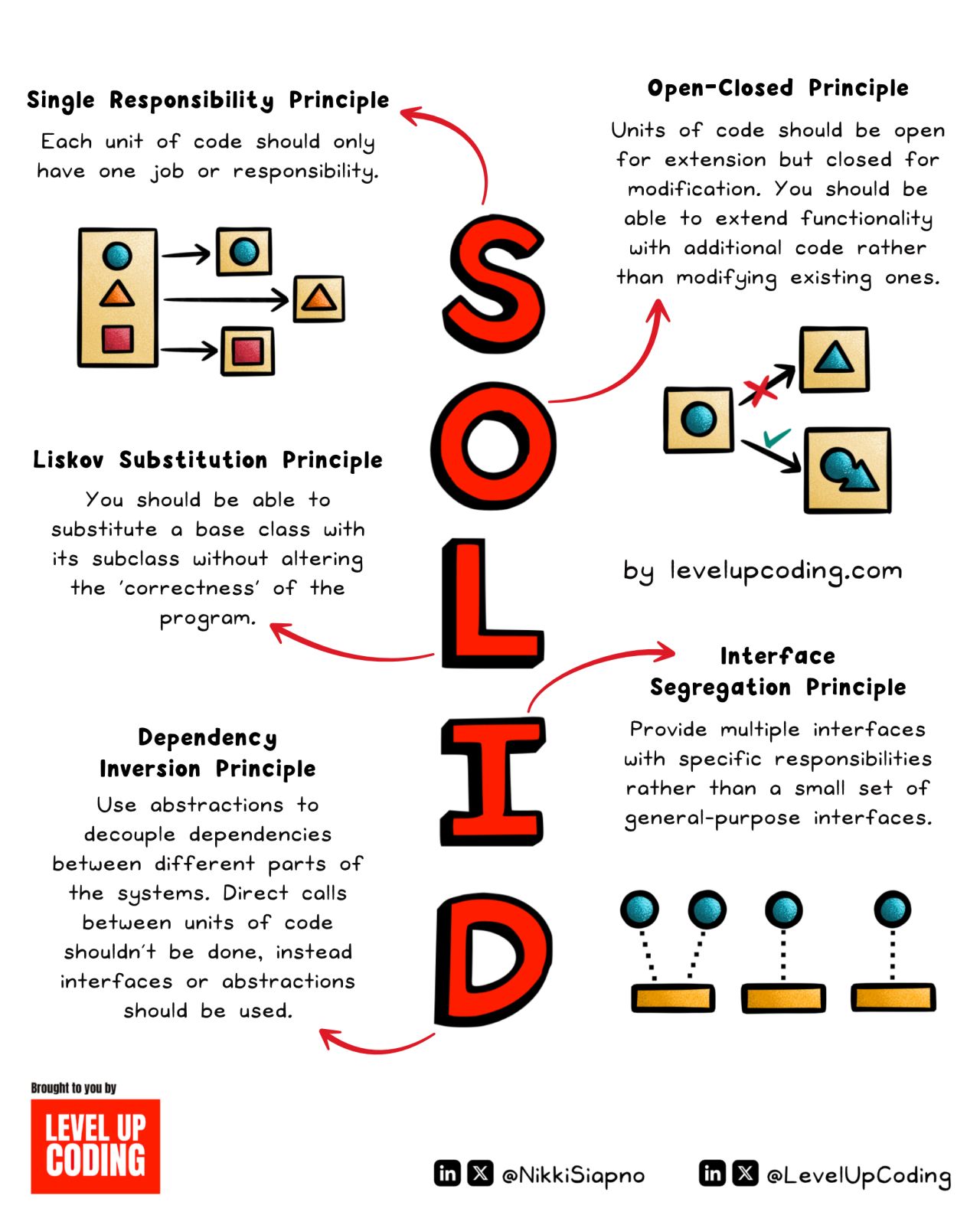
Image credits: Level up coding